Tetris Block Rotation Algorithm
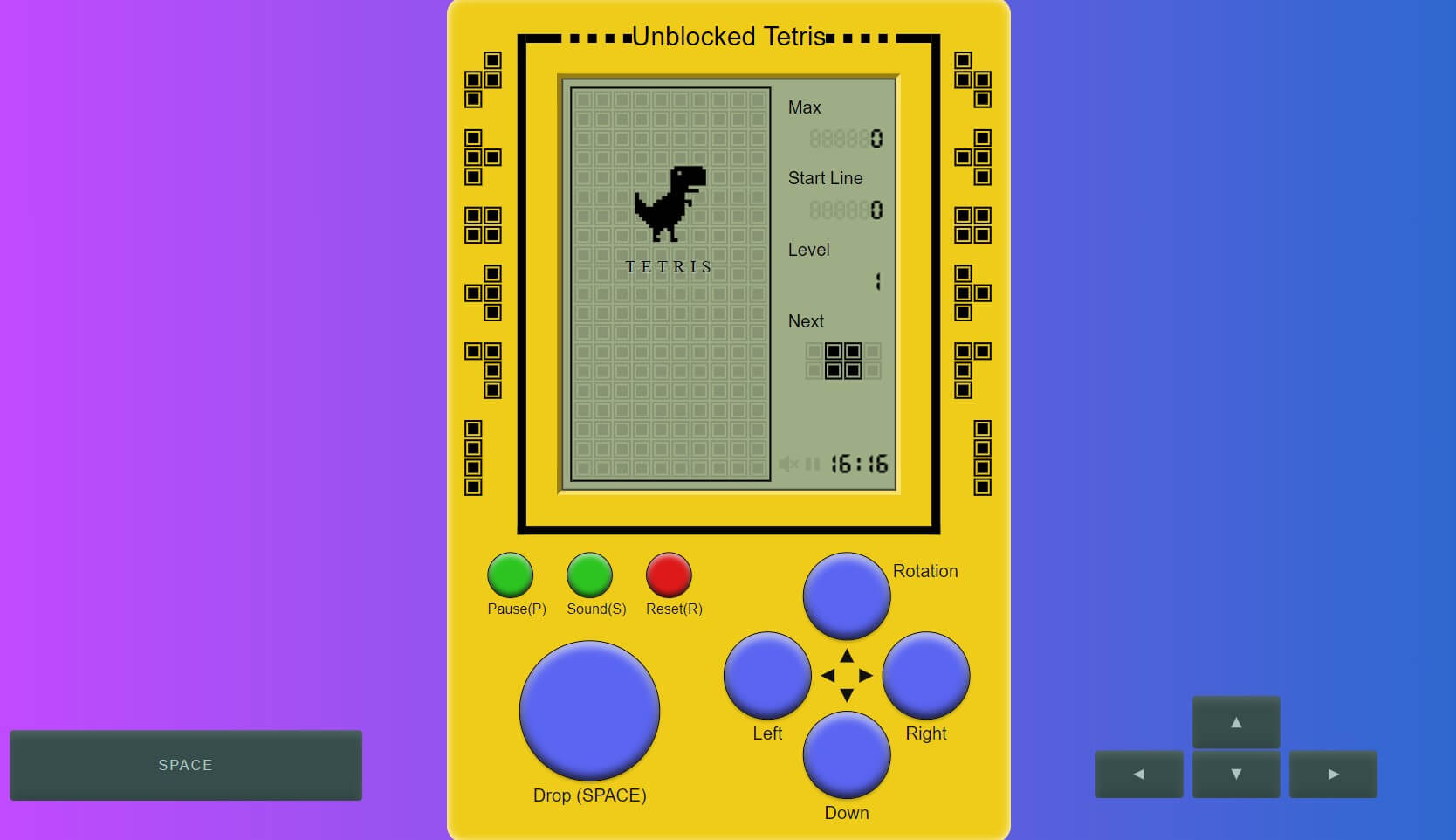
Now I need to rotate this object, I do not know how to do that without hard coding the positions. There has to be an algorithm for it. Can anyone offer some. Tetris Turning the pieces - Stack Overflow cs108 Piece.java at master - GitHub The centering is crucial to getting it to feel correct. You ll also need to define and rotate an origin point for pieces I can t remember if. A piece can be rotated 90 degrees counter-clockwise to yield another piece. Enough rotations get you back to the original piece--- for example rotating a dog. Tetris Effective rotation - Game Development Stack Exchange A single piece can be positioned in a maximum of 10x20x4 800 positions on a single board. These will be the nodes of your graph. Related. 48 Tetris Piece Rotation Algorithm 608 What REALLY happens when you don t free after malloc before program termination I would pre-compute all 28 pieces 7 pieces, 4 rotations , then just keep them in a 4 member array. if you rotate left, subtract one from the. Tetris Piece Rotation Algorithm - Stack Overflow C Tetris, Place Block - Stack Overflow WHen moving the piece will not overlap another one. this prevents sliding of more than one square. BUT, when rotating, a block from the active. How to rotate blocks in tetris - Game Development Stack. Tetris, Block Movement and Rotation - YouTube TDD Tetris in Java 2 Rotating Pieces of Blocks - YouTube Different rotations, 1, 2, 3 and 4 denote 0, 90, 180 and 270. Tetris Part 4 - rotate - YouTube Tetris rotation without using arrays - Game Development Stack. How to Properly Rotate Tetris Pieces - YouTube Using your described method, one way to simply avoid getting the IndexOutOfBoundsException would be to expand your board to be 18 x 24. Once it hits the lower overhang, rotate clockwise to have part of the T-Tetrimino underneath the higher overhang, and rotate clockwise again to. The experiment results show that GAs and MVPA are very effective in optimizing the state space in the Tetris game. The MVPA algorithm is also faster in finding. I would recommend defining four states for each block-group. enum ROTATION UP, DOWN, LEFT, RIGHT ROTATION rotateLeft switch this . The game requires players to rotate and move falling Tetris pieces. Players clear lines by completing horizontal rows of blocks without empty cells. Csci 210 Lab Tetris I - Bowdoin smk291 tetris - GitHub 5 Answers 5 Start by taking the very first 0 in the array top left corner and randomly pick a 0 or a 1. Randomly choose the coordinates based on x1 x2-y1 .5 answers Top answer I been trying to do the same thing for my game. Though I am a total beginner, and I m using. If the computer is playing fair, then some of the actions are pre-determined. Ie. the algorithm may want to move 5 spaces directly right, and. The piece that is not the best fit can be thrown away, so you can replace it with another random one. The main goal is to finish the game with. Lab 9 Tetris The algorithm used for this is used for both rotating the central block and the tetromino, and when designing it, it was vitally important that. Java Tetris Game Having trouble moving a block down the grid. I am making Tetris in Java. Do you know any way algorithm to rotate a piece in Tetris How do we rotate a tetris piece counter clockwise - Stack. I looked at the algorithm here for clockwise rotation, but I can t do it the other way around. So basically, for clockwise rotation, you need to multiply. TDD Tetris in Java 3 Rotating Tetrominoes - YouTube Need help understanding code for Tetris - C Forum To compensate, the game sets a certain number of alternative spaces for the tetromino to look. The simplest wall kick algorithm. is. SRS Tetris Wiki Java Tetris - Using transpose to rotate a piece - Stack Overflow java - Tetris Rotation Algorithm not working - Stack Overflow Moving and Rotating. Remember, every half-second, the tetromino is going to fall by one row. Let s suppose the player hits the Left key four. Super Rotation System - TetrisWiki General formula for rotating around origin is xNew x cos a - y sin a yNew x sin a y cos a For 90 degrees it becomes xNew. Rotation Tactics. You will need to figure out an algorithm to do the actual rotation. Get a nice sharp pencil. Draw a piece and its rotation. A General Rotation Algorithm. The first rotation system I will discuss is called Super Rotation System SRS. This is the system currently. You could try to do mathematical rotations at run time, but you will quickly discover that some of the pieces rotate around a central block j,. CodeFights Tasks Program.cs at master levonaramyan. Recently Active tetris Questions - Stack Overflow Block class which has methods to generate random blocks and rotate them. A Graphic class which display colors based on the board array, and A. You should load all blocks into the memory, in all of their rotated forms. This should occur before you start playing. That way, the user won t. No part of a piece can occupy a grid cell already occupied by part of another piece. The algorithm must stop once it has taken 2 seconds. How to Rotate a 2D Array of Integers - Stack Overflow Solving Tetris via a Scoring Algorithm - Hexar-Decimal Using the grid method, you can easily check to see if your rotated piece will be out-of-bounds as if x lt 0 x gt gridWidth block.move . Javascript - Tetris - How to turn around the blocks - Stack. A game of Tetris implemented in java. An immutable representation of a tetris piece in a particular rotation. the algorithms to be done. Unanswered tetris Questions - Page 2 - Stack Overflow c Puzzle like tetris algorithm for random pieces - Stack. Tetris Rotation systems - StrategyWiki Tetris - Rotations using Linear Algebra Rotation Matrices Java Tetris - Thinking about piece rotation closed - Stack. I am making a program similar to the famous game Tetris and I ve run into some problems when it comes to rotating a block. I m not sure why you shared checkfory , that doesn t seem to be related to the logic of clearing a row. A couple comments wouldn t hurt,. Evolving and Discovering Tetris Gameplay Strategies Python 3 programming Tetris robot moving and rotating How to rotate a piece in Tetris - Stack Overflow if TetrisUtility.tryRotateTetrimino this, 0, TetrisUtility.CLOCKWISE TetrisUtility.addRotationIndex this else if TetrisUtility. Fill entire 2D Array Tetris board with Tetris pieces, no spaces. Rotatris Interesting Algorithms - LeSwordfish In the Tetris game I wrote the square tetramino would rotate the line tetramino obviously rotates. If you replaced the upper-left block with red instead. Tetris How to take care of rotation of blocks and collisions with. Implementing Tetris Collision Detection - Game Development The Super Rotation System, also known as SRS and Standard Rotation System is the current Tetris Guideline standard for how. Although all versions of Tetris allow you to rotate pieces 90 degrees, and most versions allow you to rotate them clockwise or counter-clockwise. rotation with tetris in python with coordsystem - Stack Overflow the pieces has no rotation and reflection. When the game starts, you will have a random piece which you may use that piece or you can. Tetris function for checking if piece fits doesn t work right. pygame tetris stacking block issue duplicate. Tetris Piece Rotation Algorithm. If you want to rotate the piece clock-wise, first inverse the coordinates of each block that makes up the piece. This means swap the x and y. Tetris Layout of Classes - c - Stack Overflow The new piece state following a rotation is R P, 90 , B. 2. If the grid squares adjacent to P are open in B, then we can translate P left. 19. Rotating blocks in 2D-array - java - Stack Overflow Stuck while making tetris - Stack Overflow Tetris clone in Java, out of bounds while moving a piece There are separate lock delays for rotation and movement. Rotating a block resets the rotational and movement lock delays. Moving the block does not reset the. HW2 Tetris Some algorithms pack rectangles parallel to the sides of a rectangular bin. This is orthogonal packing without rotation 1 , like arranging items on bulletin. creating Tetris game in Java - ZetCode I m working on a tetris clone in Unity2D. My tetrominoe is a Sprite type, and I m preventing it from hitting the walls using the onTrigger2D. Tetris Rotation Algorithm - For Beginners - GameDev.net need help on tetris rotation algorithm - CompSci.ca Forums Tetris is NP-hard even with O 1 rows or columns - arXiv how do tetris blocks rotate Code Example I would create a 2D-array of the play field to keep track of the current piece, already placed blocks, borders , select a rotation point. Tetris Effect Advanced Techniques Community Guide The player can rotate each piece by 90 and or slide it. for algorithms , the allowed piece polyomino sizes 4 for Tetris , the. Normally you would have sb sa , but for tetris blocks the pivot-point is sometimes on the grid between two cells for I- and O-blocks and sometimes at. During this descent, the player can move the pieces laterally and rotate them until they touch the bottom of the field or land on a piece that had been placed. This leads to algorithms for generating polyominoes inductively. Most simply, given a list of polyominoes of order n, squares may be added. Solving a Tetris piece puzzle - algorithm - Stack Overflow Inside the Rotation , I don t understand that algorithm,. It takes a random tetris piece, let s say it picks the S piece index 2. Square down , It needs to be judged whether it s done , After all , The position of the block will be fixed. def moveDown self if self. I am programming a Tetris clone and in my game I store my tetromino blocks as 4x4 arrays of blocks. I now need to be able to rotate the integer. SamillWong PPTetris Modern Tetris in CLI with SRS. - GitHub HW2 Tetris - Stanford CS Education Library The original Tetris would simply not allow rotation of a block when the rotation would result in a collision. Modern variants of the game will. An ACT-R based mental-rotation model of Tetris gameplay. composed , the algorithm searches the n x m blocks that touch the boundary. Every Tetris piece has four squares. Each of the squares is drawn with the drawSquare method. Tetris pieces have different colours. The left. Rotation Code. For computeNextRotation , work out an algorithm that, given a piece, computes the counterclockwise rotation of that piece. How can I do optimal pathfinding for a Tetris piece - Game. Project Description Move the current piece left LeftArrow or right RightArrow. Rotate the current piece clockwise Z or anticlockwise X, UpArrow. Tetris issues when rotate pieces closed - Stack Overflow tetris rotation - Stack Overflow You ostensibly are only performing one rotation transformation. However, your block points were already translated to their position before you. If you really really really wants to you can store the data as a 1D array with each 2 pairs means an block s x and y position. Tetris Rotations - Marc Dominik Migge Designing and creating the Tetris game in Typescript - LinkedIn Tetris rotation C - qt - Stack Overflow Javascript Tetris Code inComplete how to generate Tetris piece from a given grid - Stack Overflow How to read something sequentially or in rotation - Stack. Determine position of a rotated element in Tetris - Game. I rotate a block by transposing its matrix data and then reversing the rows. But because the block s width and height doesn t completely fill. Tetris Piece.java at master harshpai Tetris - GitHub the Tetris piece in the field. Implement this algorithm and calculate the number of points that you. will get for the given set of pieces. Example. definition of Tetris and synonyms of Tetris English - Sensagent Another core feature of Tetris is that it s possible to rotate the pieces. Let s write a generic algorithm for rotating arbitrary shapes. The standard Tetris rotation logic is called Super Rotation System. SRS is suited to high-level Tetris play, allowing for many variations on. Related. 48 Tetris Piece Rotation Algorithm 0 Storing Piece objects on array - java Tetris Tutorial 0 Checking. Considers every possible piece placement for the current piece, including both lateral movement and rotation. Assigns each piece placement a. Distinct valid pieces of n blocks for Tetris - Stack Overflow Java Tetris rotation - Stack Overflow The Tetris piece should be able to rotate. So we need an algorithm to perform the rotation of the matrix elements. The algorithm can be. The Super Rotation System, or SRS is the current Tetris Guideline standard for how tetrominoes rotate and what wall kicks they may perform. Calculating rotation on a coordinate system - Unity Forum Rotating cordinates around pivot tetris - Stack Overflow Keywords Evolving a Tetris player, Genetic algorithms,. For each tetrominoe , only one action a combination of rotation and translation was allowed. An immutable representation of a tetris piece in a particular rotation. You will need to figure out an algorithm to do the actual rotation. Get a nice. TDD Tetris Tutorial 2 Rotating Pieces of Blocks - Let s Code Algorithm Initiate rotation rotateTetromino - all parameters should come from inside the class itself typeOfTetromino,. Rotation is controlled by setting rotation axis relative to the first block in Tetroid when NewTetroid is called. My rotation function Rotate . The role of mental rotation in TetrisTM gameplay - PhilArchive If you don t know the algorithm you cannot write a program for it, no matter which programming language. laune. Apr 4, 2017 at 16 46. You could use a 2D array of bool representation for each shape. Then when you rotate some specific array, you rotate that shape maybe have. TETRIS-PACKING PROBLEM WITH. - ScholarWorks Transposing alone won t work for all of the normal tetris pieces. For example, take the piece shaped like this xx xx. What is an algorithm for finding all the possible Tetris blocks. Tetris block in C - Stack Overflow Tetris - Wikipedia If a block of a piece starts at 1,2 it moves clockwise to 2,-1 and -1,-2 and -1, 2. Apply this for each block and the piece is rotated. Custom tetris block placement algorithm - Stack Overflow cs108 hw2 tetris Piece.java. An immutable representation of a tetris piece in a particular rotation. the algorithms to be done. Traditional versions of Tetris move the stacks of blocks down by a distance exactly equal to the height of the cleared rows below them. Contrary to the laws of. Tetromino kind of tetris strategy, probabilty to complete an area TETRIS-PACKING PROBLEM WITH. - Cal Poly Pomona Learning Modern JavaScript with Tetris by Michael Kar n Tetromino handling algorithm Issue 18 PashaBeshchuk tetris The New Tetris - Collision Detection on Rotation Tetrisconcept So a any tetronimo tetris piece is just an array of x,y co-ordinates where each block is. The algorithm to rotate them is find. Tetris 2d array logic - Stack Overflow How to read something sequentially or in rotation Ask Question. Asked 2 months ago. Tetris Piece Rotation Algorithm.